HTTP 1.0 introduced the idea of content encodings. A browser/client can notify the server that it can accept compressed content by sending the Accept-Encoding header. The Accept-Encoding header can be set as follows
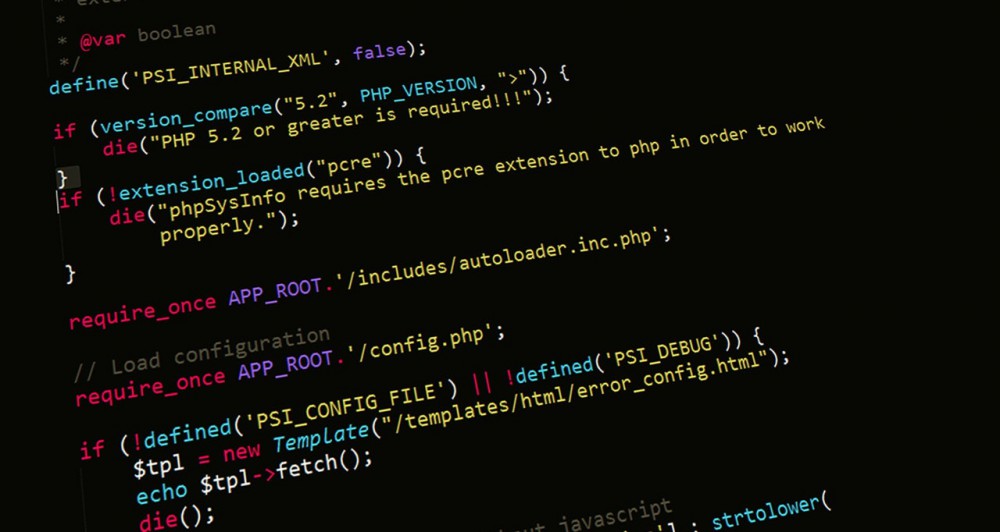
Accept-Encoding: gzip,deflate
or with just one of gzip or deflate. You don't need to worry about any of this - PHP will automatically choose the correct compression to use.
Compressing content usually results in less data being send across the network. This has three benefits:
- Bandwidth usage decreases - you can serve more visitors before you reach your bandwidth limit
- Network latency can be reduced because fewer packets will be used to transmit the data. Your application can appear faster to a user - page load times are reduced.
- compressing files allows you to format your xhtml code for readibility without sacrificing page load times.
There are two methods to using compression in PHP:
Using zlib.output_compression
Your PHP needs to be built with the zlib extension. In your php.ini file set
zlib.output_compression = On
to enable compression. You also need to make sure zlib.output_handler is empty.
You can change the level of compression by using
zlib.output_compression_level = d
where d is a digit from 1(minimal compression) to 9(maximum compression). The higher compression levels increase CPU usage, possibly without any significant reduction in content size. Probably best to use a midrange value like 5. Or you can test different values and compare the content compressions. whatsmyip.org/mod_gzip_test shows you the original and compressed content sizes for any page you submit. It also tells you if your content is compressed - good for checking if your settings are working.
The PHP manual recommends using this method over the next method.
Note: You can only enable zlib.output_compression in your php.ini file, even though the PHP documentation suggests otherwise.
Using ob_start
The disadvantage with zlib is you need access to the php.ini file, if zlib.output_compression is not already enabled (phpinfo() can show you if its enabled). Some ISPs do not give you access to the php.ini file.
You can use PHP's output buffering to compress your pages. In your scripts, or on the page you want compressed, add the following line
ob_start('ob_gzhandler');
This should be at the very top of your scripts. It needs to be called before any content is send to the browser/client. If you have an include file that you use for all your pages, you might want to put this line at the top of that file.
This will put all output in PHP's output buffer. At the end of the script, the function ob_gzhandler is called. This callback function determines the compression to use ('deflate' or 'gzip'), and compresses the content. The compressed content is then send to the browser/client.
Conclusion
You have seen two methods of compressing content using PHP with very little effort on your part. I have seen page sizes reduced by up to 68% using these methods. Not bad for one line of code!
For more on the Accept-Encoding header, follow the link.